728x90
반응형
이번엔 진짜 혼자 풀었다!!
이제 recursive 알고리즘의 개미 발톱은 보이는 것 같다.!
문제
이번 문제는 root의 자식 노드 중 leaf 노드를 찾는 것!
여기서 leaf 노드란 ?
자식이 없는 노드를 뜻함.!
여기서 약간의 함정이 있다고 보는데,,..
내가 생각하는 함정은 root의 자식이 없을 경우이다.
이것때문에.. 힘들었즤.. 하하
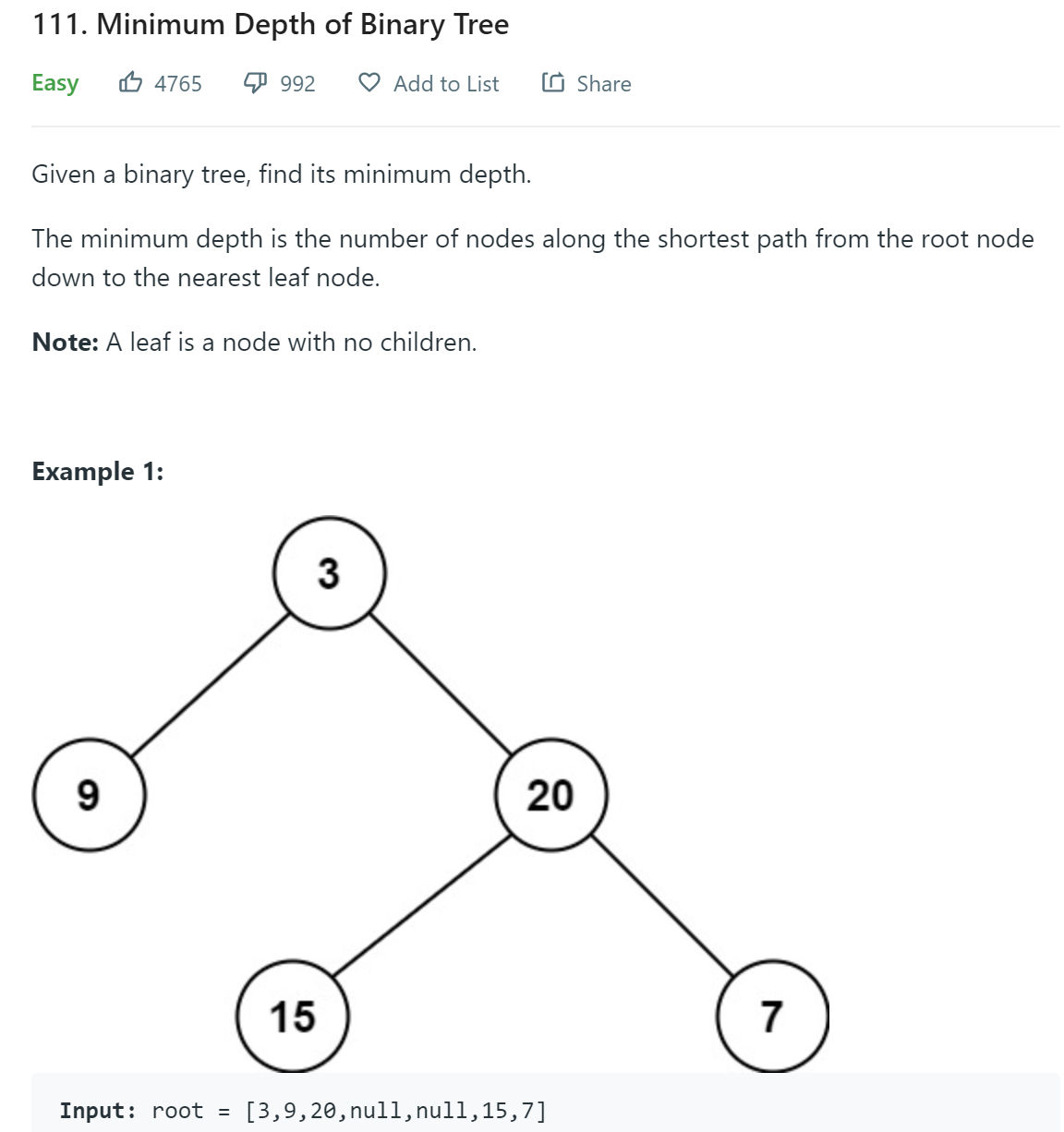
나의 오답
창피하지만.. 내 오답을 적어놔야 나중에 실수를 안하니깐!
/**
* Definition for a binary tree node.
* public class TreeNode {
* int val;
* TreeNode left;
* TreeNode right;
* TreeNode() {}
* TreeNode(int val) { this.val = val; }
* TreeNode(int val, TreeNode left, TreeNode right) {
* this.val = val;
* this.left = left;
* this.right = right;
* }
* }
*/
class Solution {
public int minDepth(TreeNode root) {
if(root == null) return Integer.MAX_VALUE;
if(root.right == null && root.left == null) return 1;
return Math.min(minDepth(root.right)+1, minDepth(root.left)+1);
}
}
나는 자식 노드가 둘 다 없는 경우만 고려하고, 나머지는 주인공 노드로 생각하고 구현했음..

그니까 이럴 경우에 right가 될 경우 MAX를 보내면 생각 안하겠거니 했는데,..
시작 노드(root) 가 null 일 경우에도 이 값을 return 해서 틀렸다.
그래서 root 가 null 일 때랑
자식 노드 중 한 가지가 null일 경우를 다르게 생각해줘야 한다는 것을 깨달았음!
그래서 나온
내 정답
/**
* Definition for a binary tree node.
* public class TreeNode {
* int val;
* TreeNode left;
* TreeNode right;
* TreeNode() {}
* TreeNode(int val) { this.val = val; }
* TreeNode(int val, TreeNode left, TreeNode right) {
* this.val = val;
* this.left = left;
* this.right = right;
* }
* }
*/
class Solution {
public int minDepth(TreeNode root) {
if(root == null) return 0;
if(root.right == null && root.left == null) return 1;
int rightDepth = Integer.MAX_VALUE;
int leftDepth = Integer.MAX_VALUE;
if(root.right != null) rightDepth = minDepth(root.right) + 1;
if(root.left != null) leftDepth = minDepth(root.left) + 1;
return Math.min(rightDepth, leftDepth);
}
}
이렇게 따로 생각해줘야하는구나!!
간단하게만 풀려고 하다보니 틀려버리고 말았구나!!!
간단하게만 하려고하지말고 다음엔 정확하게 풀려고 노력하기!!!

진짜 이제 내 힘으로 푼 문제가 나오다니..
후후
recursive 마스터 하고 말겠어..!!
근데 생각해보니 이것 .. EASY 문제이다 .. 하하하하핳!!!
나의 손코딩 흔적 기록
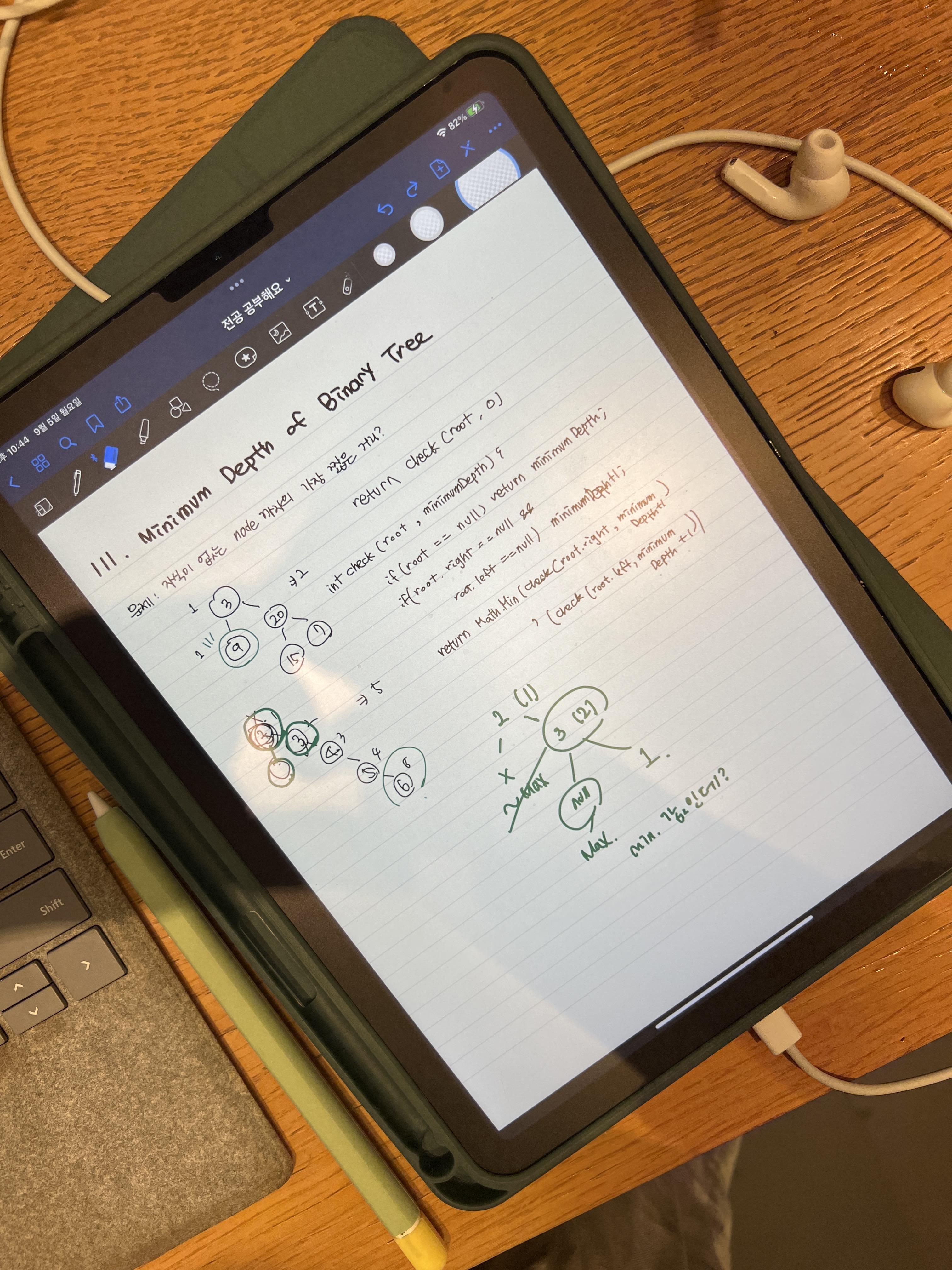
728x90
반응형
'ALGORITHM > Java algorithm' 카테고리의 다른 글
[JAVA][LeetCode][BST] #98. Validate Binary Search Tree (0) | 2024.04.09 |
---|---|
[JAVA][LeetCode] #1. Two Sum (1) | 2022.11.21 |
[LeetCode][Java] 110. Balanced Binary Tree (0) | 2022.09.05 |
[LeetCode][Java] 101. Symmetric Tree (0) | 2022.09.01 |
[LeetCode][Java] 100. Same Tree (0) | 2022.09.01 |